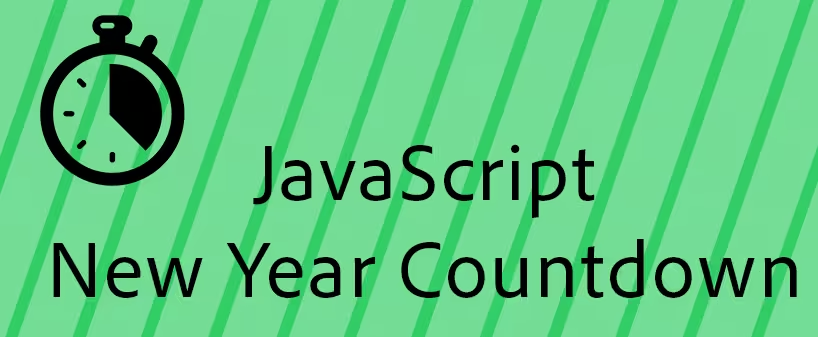
Create a countdown timer in JavaScript
A countdown timer is a simple yet practical feature for many applications, such as event countdowns, task deadlines or games. This blog post will guide you through creating a functional countdown timer using HTML, CSS and JavaScript.
This example features a countdown timer for New Year’s Eve but it can be easily adapted to your needs. I’m using JavaScript functionality to detect current date and time on page load and set timer to countdown till New Year’s Eve.
Demo
0
Days0
Hours0
Minutes0
Seconds
In this tutorial, we will focus on the JavaScript part of creating a countdown timer. We will use JavaScript to get current time and date to calculate the time remaining until a specified date and time (New Year’s Eve) and update the timer dynamically. This will involve working with JavaScript’s Date object, manipulating the DOM to display the timer and using setInterval to update the timer every second.
HTML
HTML code snippet below creates a countdown timer with a structured layout.
Here’s a breakdown of its components:
An <h2> element with the class=”text-center” and the id=”today-date” is used to display the current date at the top of the timer.
A <div> with the id=”counter-block” and several CSS classes (border, border-2, border-green, rounded-3) serves as the container for the countdown timer. This container has a border and rounded corners thanks to classes above.
Inside the id=”counter-block” there are four divs with class=”counter-item” each representing a different time unit (Days, Hours, Minutes, Seconds):
Each div with class=”counter-item” contains an <h2> element with an ID (counter-days, counter-hours, counter-minutes, counter-seconds) initialized to 0, which will display the countdown values.
Each div with class=”counter-item” also includes a element to label the time unit (Days, Hours, Minutes, Seconds).
Finally, there is a <div> with the id=”counter-end” containing an <h3> element, which can be used to display a message when the countdown ends.
This structure provides a clear and organized way to display a countdown timer on a webpage.
<h2 class="text-center" id="today-date"></h2>
<div id="counter-block" class="border border-2 border-green rounded-3">
<div class="counter-item">
<h2 id="counter-days">0</h2>
<span>Days</span>
</div>
<div class="counter-item">
<h2 id="counter-hours">0</h2>
<span>Hours</span>
</div>
<div class="counter-item">
<h2 id="counter-minutes">0</h2>
<span>Minutes</span>
</div>
<div class="counter-item">
<h2 id="counter-seconds">0</h2>
<span>Seconds</span>
</div>
<div id="counter-end">
<h3></h3>
</div>
</div>
CSS
The CSS code used for styling the countdown timer block is mostly custom. Note: I use https://getbootstrap.com/, a CSS framework for developing responsive and mobile-first websites, so some of the styling is applied from there.
This CSS code also ensures that the countdown timer block is responsive and well-styled across different screen sizes.
#counter-block {
display: flex;
justify-content: space-around;
flex-direction: column;
align-items: center;
}
@media(min-width: 769px) {
#counter-block {
flex-direction: row
}
}
#counter-block .counter-item {
display: flex;
justify-content: center;
flex-direction: column;
}
@media(min-width: 901px) {
#counter-block .counter-item {
margin: 0 20px;
width: 160px;
}
}
#counter-block h2 {
text-align: center;
font-size: 50px;
font-style: normal;
font-weight: 600;
line-height: 100px;
margin: 0;
}
@media(min-width: 901px) {
#counter-block h2 {
font-size: 70px;
line-height: 130px;
}
}
#counter-block h3 {
text-align: center;
font-size: 40px;
font-style: normal;
font-weight: 600;
line-height: 60px;
margin: 0;
}
@media(min-width: 901px) {
#counter-block h3 {
font-size: 50px;
line-height: 70px;
}
}
#counter-block span {
text-align: center;
font-size: 20px;
font-style: normal;
font-weight: 400;
line-height: normal;
margin-bottom: 30px;
}
#counter-block #counter-end {
display: none;
}
JavaScript
Here is a breakdown of JavaScript code in simple way explaining every line.
1. Current Date and Time
const currentDate = new Date(); // Get the current date and time
const currentDayDate = currentDate.getDate(); // Get the day of the month (e.g., 1–31)
currentDate creates a Date object representing the current date and time.
currentDayDate extracts the numeric day of the month (e.g., 1 for the 1st, 2 for the 2nd).
2. Day and Month Names
const dayNames = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
const currentDayName = currentDate.getDay(); // Get the index of the current day (0 = Sunday, 6 = Saturday)
const monthNames = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
const currentMonthName = monthNames[currentDate.getMonth()]; // Get the name of the current month
dayNames and monthNames are arrays that map day and month indices to their names.
currentDayName and currentMonthName fetch the current day and month names using getDay() and getMonth().
3. Year and Next Year
const currentYear = currentDate.getFullYear(); // Get the current year
const nextYear = currentYear + 1; // Calculate the next year
The currentYear is determined using getFullYear().
The nextYear is calculated by adding 1.
4. Display Today’s Date
document.getElementById("today-date").innerHTML = "Today is " + dayNames[currentDayName] + " , " + currentDayDate + " " + currentMonthName + " " + currentYear;
This updates an HTML element with id=”today-date” to display the current date in a readable format like: “Today is Tuesday, 12 December 2023.”
5. Countdown Timer
const endDate = [currentYear, 11, 31, 24, 00, 00, 00];
var countDownDate = new Date(...endDate).getTime();
endDate is an array representing December 31, midnight of the current year.
The new Date(…endDate).getTime() converts it into a timestamp (milliseconds since January 1, 1970).
6. Countdown Logic
var countdown = setInterval(function() {
var now = new Date().getTime(); // Current timestamp in milliseconds
var timeleft = countDownDate - now; // Remaining time in milliseconds
....
}, 1000);
setInterval runs the function every second (1000 ms).
The remaining time (timeleft) is calculated as the difference between countDownDate and the current time (now).
7. Time Breakdown
var days = Math.floor(timeleft / (1000 * 60 * 60 * 24)); // Days left
var hours = Math.floor((timeleft % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)); // Hours left
var minutes = Math.floor((timeleft % (1000 * 60 * 60)) / (1000 * 60)); // Minutes left
var seconds = Math.floor((timeleft % (1000 * 60)) / 1000); // Seconds left
The remaining time is broken down into:
Days: Total remaining time divided by milliseconds in a day.
Hours, Minutes, Seconds: Calculated using the remainder (%) of the higher unit.
8. Update Countdown in HTML
document.getElementById("counter-days").innerHTML = days;
document.getElementById("counter-hours").innerHTML = hours;
document.getElementById("counter-minutes").innerHTML = minutes;
document.getElementById("counter-seconds").innerHTML = seconds;
Updates the HTML elements with id=”counter-days”, id=”counter-hours”, etc., to display the live countdown.
9. Countdown End
if (timeleft <= 0) {
clearInterval(countdown); // Stop the interval timer
// Reset the countdown display to 0
document.getElementById("counter-days").innerHTML = "0";
document.getElementById("counter-hours").innerHTML = "0";
document.getElementById("counter-minutes").innerHTML = "0";
document.getElementById("counter-seconds").innerHTML = "0";
setTimeout(function() {
var counterItem = document.getElementsByClassName('counter-item');
for (var i = 0; i < counterItem.length; i ++) {
counterItem[i].style.display = 'none'; // Hide countdown items
}
document.getElementById("counter-end").style.display = "block"; // Show the "Time is up" message
document.getElementById("today-date").style.visibility = "hidden"; // Hide the today's date
document.getElementById("counter-end").querySelector('h3').innerHTML = "Time is up,<br>Happy New Year " + nextYear;
}, 1000);
}
If timeleft is <= 0:
The countdown stops (clearInterval).
The countdown display resets to 0.
After a 1-second timeout, the script hides the countdown elements and displays a “Time is up, Happy New Year [Next Year]” message.
Complete JavaScript code below
const currentDate = new Date();
const currentDayDate = currentDate.getDate();
const dayNames = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"];
const currentDayName = currentDate.getDay();
const monthNames = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December'];
const currentMonthName = monthNames[currentDate.getMonth()];
const currentYear = currentDate.getFullYear();
const nextYear = currentYear + 1;
const endDate = [currentYear, 11, 31, 24, 00, 00, 00];
document.getElementById("today-date").innerHTML = "Today is " + dayNames[currentDayName] + " , " + currentDayDate + " " + currentMonthName + " " + currentYear;
var countDownDate = new Date(...endDate).getTime();
var countdown = setInterval(function() {
var now = new Date().getTime();
var timeleft = countDownDate - now;
var days = Math.floor(timeleft / (1000 * 60 * 60 * 24));
var hours = Math.floor((timeleft % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
var minutes = Math.floor((timeleft % (1000 * 60 * 60)) / (1000 * 60));
var seconds = Math.floor((timeleft % (1000 * 60)) / 1000);
document.getElementById("counter-days").innerHTML = days;
document.getElementById("counter-hours").innerHTML = hours;
document.getElementById("counter-minutes").innerHTML = minutes;
document.getElementById("counter-seconds").innerHTML = seconds;
if (timeleft <= 0) {
clearInterval(countdown);
document.getElementById("counter-days").innerHTML = "0";
document.getElementById("counter-hours").innerHTML = "0";
document.getElementById("counter-minutes").innerHTML = "0";
document.getElementById("counter-seconds").innerHTML = "0";
setTimeout(function() {
var counterItem = document.getElementsByClassName('counter-item');
for (var i = 0; i < counterItem.length; i ++) {
counterItem[i].style.display = 'none';
}
document.getElementById("counter-end").style.display = "block";
document.getElementById("today-date").style.visibility = "hidden";
document.getElementById("counter-end").querySelector('h3').innerHTML = "Time is up,<br>Happy New Year " + nextYear;
}, 1000);
}
}, 1000);
How it works in action
-
JavaScript displays today’s date and shows a live countdown to December 31 at midnight on page load.
-
The countdown elements are hidden once the countdown ends.
-
A “Happy New Year” message is displayed.
When you reload the page in the new year, JavaScript will start countdown to current year.
Feel free to get in touch by visiting https://josefzacek.com/contact/ if you would like to integrate something like this into your project or website.