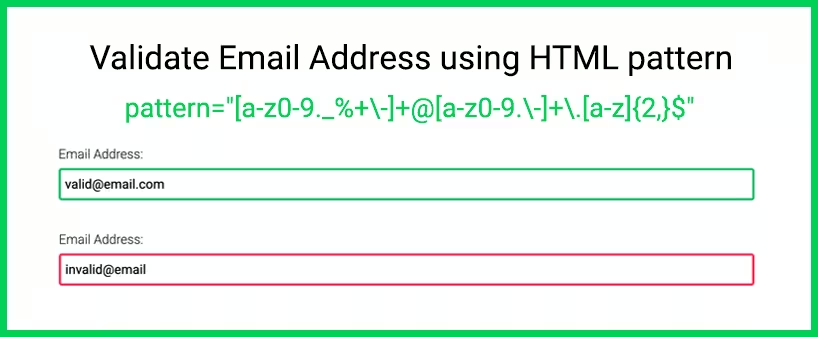
How to Validate Email Address using HTML pattern
Validating an email address on the frontend helps improve user experience and reduce errors. HTML provides simple, built-in ways to validate email formats using the type="email"
attribute and regular expressions via the pattern
attribute.
1. Basic HTML Email Input
The easiest way to validate an email is to use the type="email"
input:
<input type="email" required />
This tells the browser to expect an email format (e.g. info@josefzacek.com
).
2. Using a Custom Pattern
To apply stricter rules, add a pattern
attribute with a regular expression:
<input
type="email"
required
pattern="[a-z0-9._%+\-]+@[a-z0-9.\-]+\.[a-z]{2,}$"
placeholder="info@josefzacek.com"
title="Please enter a valid email address" />
<input
- Starts the HTML input tag, used for form fields.
type="email"
- Specifies the input type as an email field.
required
- Makes the field mandatory, the form cannot be submitted if this field is empty.
pattern="[a-z0-9._%+\-]+@[a-z0-9.\-]+\.[a-z]{2,}$"
- Adds a regular expression for custom validation.
This pattern allows:
-
Lowercase letters a-z
-
Digits 0-9
-
Characters ._%+- in the local part (before @)
-
Dashes and dots in the domain part
-
A top-level domain with at least 2 letters
placeholder="info@josefzacek.com"
- Shows a sample value in the field when it’s empty.
title="Please enter a valid email address"
- Provides a custom tooltip message that appears on hover.
/>
- Closes the <input>
tag (self-closing since it’s an empty element).
This pattern ensures the email includes lowercase letters, numbers and special characters and ends with a domain like .ie
or .com
.
3. Live Feedback with CSS
We can add CSS to visually indicate if the email input is valid or not:</p>
input:invalid {
border: 3px solid #ff3860;
}
input:valid {
border: 3px solid #09c372;
}
4. Demo
Try typing an email address in the input below. Once you click outside the input the validation will trigger automatically.
Conclusion
When building forms, ensuring users enter valid email addresses is essential for maintaining clean data and improving communication. Here’s what to keep in mind:
-
Use
type="email"
for built-in validation. This is the easiest and most effective way to ensure the email field contains an @ symbol and a valid domain. Most modern browsers also offer helpful UI features like auto-suggest. -
Add a
pattern
only if you need stricter rules. Whiletype="email"
provides basic validation, you can use a custompattern
attribute if you want to enforce stricter rules. -
Always validate email addresses on the server as well. Frontend validation is for user convenience and preventing obvious mistakes. For real data integrity, make sure your server-side logic validates the email format too.